Intro
Go, a statically typed compiled language often described as C for the 21st century. It's a popular choice for high-perfomance server-side applications and is a language that built tools like Docker, CockroachDB, Dgraph.
Go was developed at Google by Robert Griesemer, Rob Pike, and Ken Thompson in 2007.
Go was designed for simplicity and efficiency. It generally outperforms interpreted languages and has extremely fast compile times.
Go also has a package and module system making it easy to import and export code between projects.
Installation
Pretty simple, you can go to Go official website, and follow the instruction
Some basic syntax
Line Separator
In a Go program, individual statements don't need a special separator like ;
in C or Java. The line separator key is a statement terminator.
Example:
fmt.Println("Hello, World!")
fmt.Println("I am in Go Programming World!")
Comments
Single-line comments start with two forward slashes (//
)
Multi-line comments start with /*
and ends with */
.
Example:
/* This is a comment */
Identifiers
A Go identifier starts with a letter A to Z or a to z or an underscore _
followed by zero or more letters, underscores, and digits (0 to 9).
Go does not allow punctuation characters such as @
, $
, and %
within identifiers.
Go is a case-sensitive programming language.
Some acceptable examples:
abc abcdef abc123 abc abcDEF abc123
Keywords
Here are the reserved words that may not be used as constant or variable or any other identifier names.
break | default | func | interface | select |
case | defer | Go | map | Struct |
chan | else | Goto | package | Switch |
const | fallthrough | if | range | Type |
continue | for | import | return | Var |
Hello World program
1. First, using Visual Code or any code editor, create a file with .go
extension.
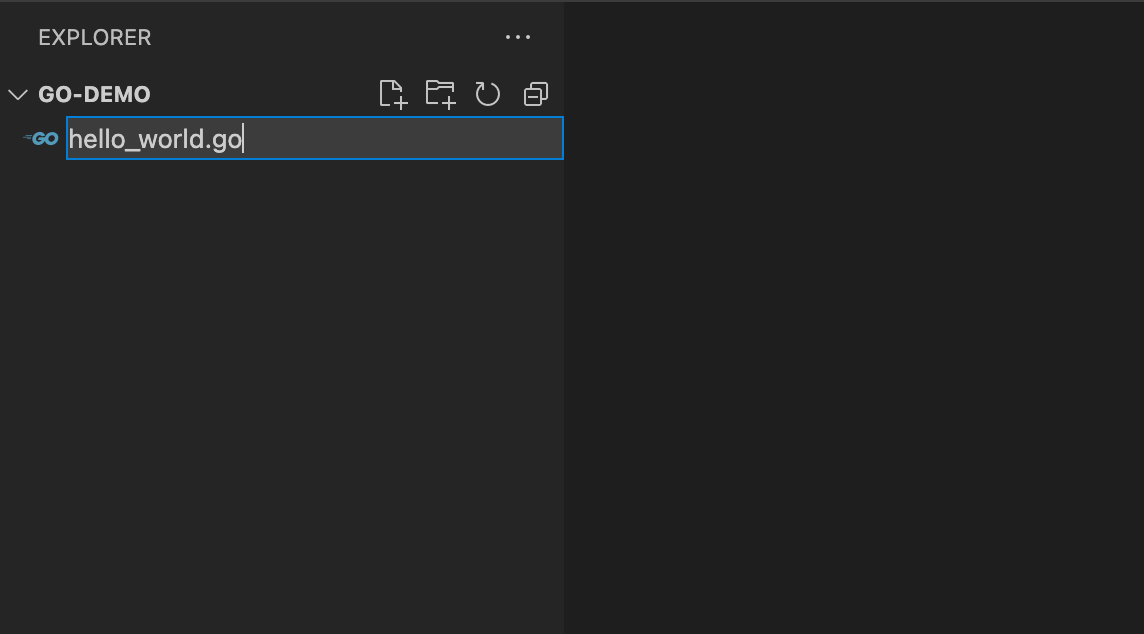
2. Then, add package main
into the file.
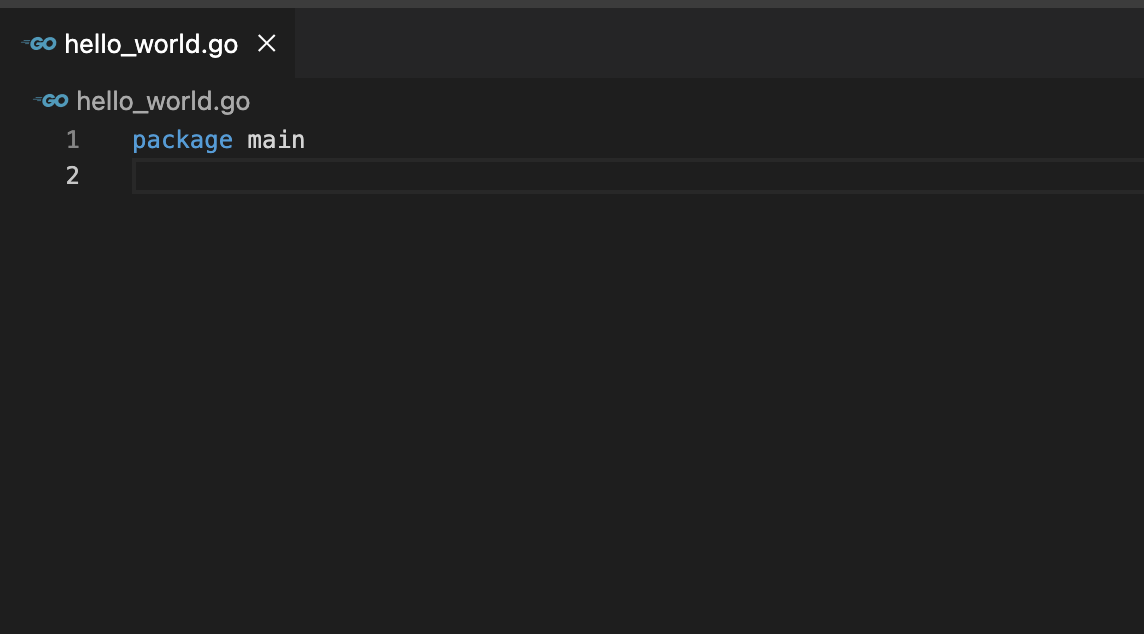
This will be the starting point of the program.
3. Next, import fmt
package.
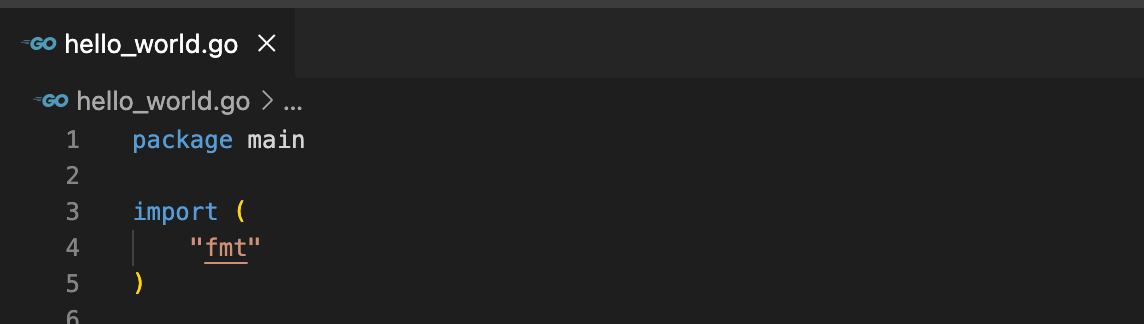
fmt
is Format package. It implements formatted I/O with functions analogous to C's printf and scanf.
4. Now write this code after the import
func main() {
fmt.Println("!... Hello World ...!")
}
In the above lines of code we have:
- func: a keyword used for creating function
- main: main function in Go language, which doesn’t take any parameter or return anything, and it is the entry point of the executable programs.
- Println(): this method is from
fmt
package, it is used to display “!… Hello World …!” string. Println means Print line.
5. Run the program. By now, your file should look something like this
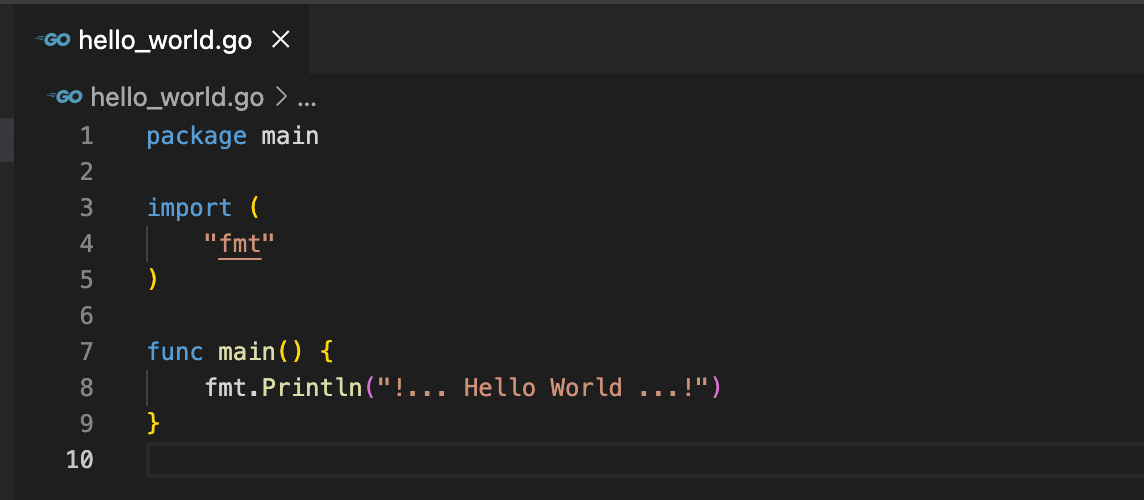
Open Terminal
(Mac or Linux) or Cmd
(Windows) or click on Terminal
tab in Visual Code. Navigate to your project folder, and run command:
go run <your_file_name>.go
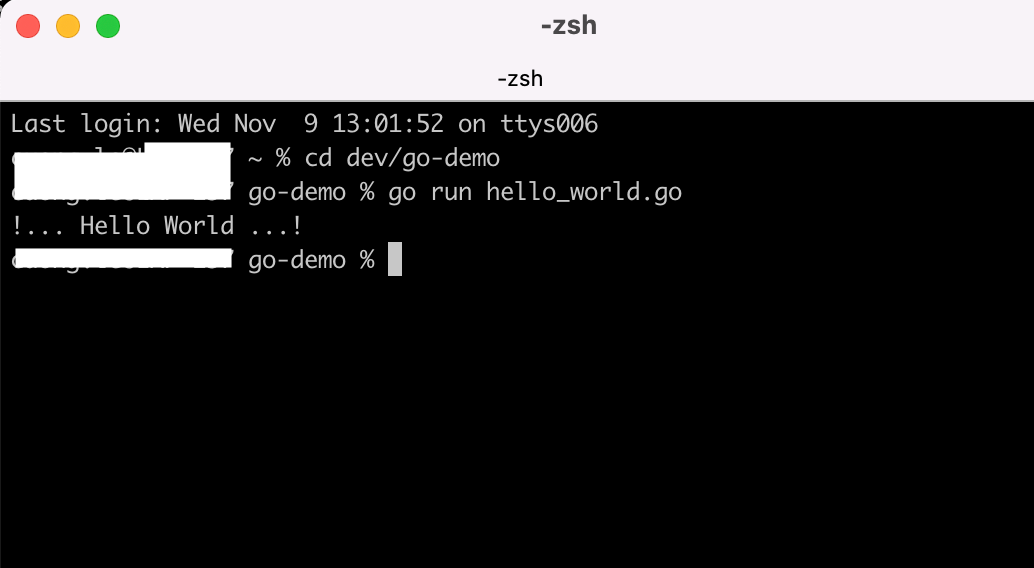
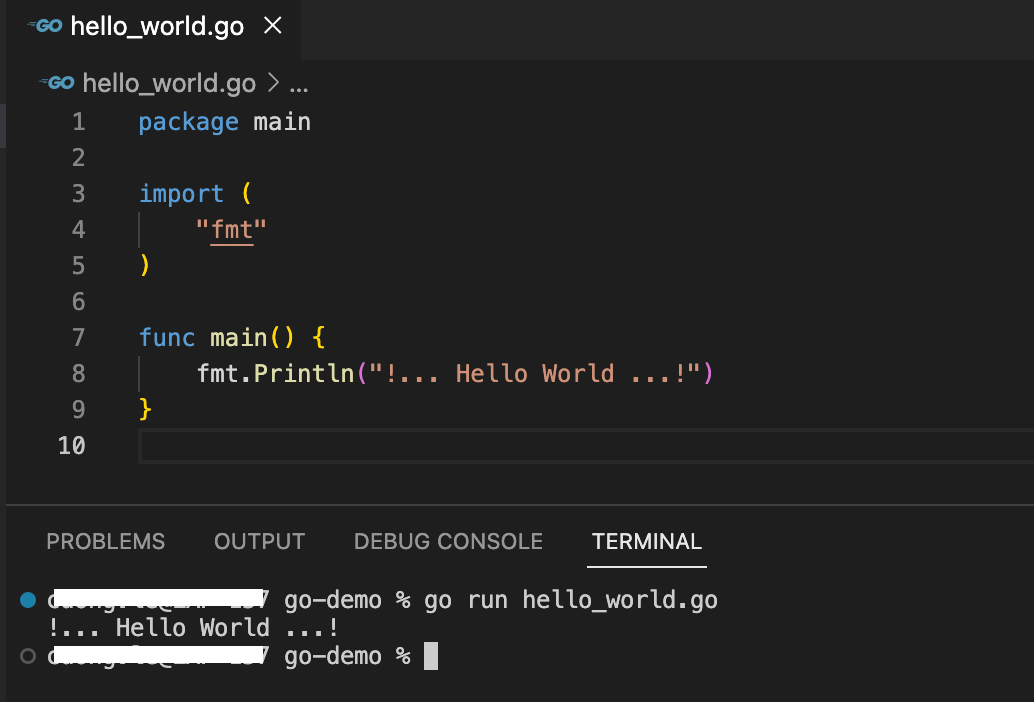
That's all. Thanks for reading.
Resources to learn more:

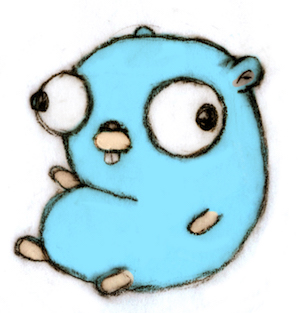
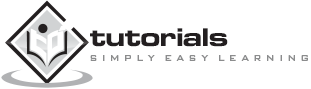