Hi you all, surely we have encountered many times after the code fix is deployed then client gives feedback that some other features no longer works correctly. There might be reasons like we just only focus testing on the code changes and forget to re-verify other related features (or maybe we're quietly confident that there will be no problems this time :D).
Therefore to ensure code quality we usually apply unit tests, integration tests and end-to-end testing:
- Unit Tests: Unit tests are the easiest tests to write because you can expect specific results for your input. There are no dependencies or complex interactions.
- Integration Tests: Integration tests are more complex than unit tests because you have to deal with dependencies.
- End-To-End: End-to-end tests simulate a specific user interaction flow with your app. For example, clicking or entering text.
Unit Tests and Integration Tests are probably familiar to you so I skip them in this scope, about End-to-End (e2e) testing I saw very few projects I joined apply it, but honestly it brings the great value:
- Simulate how users interact with the website with different flows
- If written well, test scenario can be a good document for new members joining the project
- Make sure the behaviors in flow are always consistent
To apply e2e testing we need the support of a tool/framework and they should have the following criteria:
- Clear document.
- Easy to write test scenario: provide commands, APIs, utilities that help writing specs fast and simple.
- Easy to run tests and visualize each step in the test.
- Easy to integrate with CI tools.
- Allow take screenshot or video record for reviewing later.
After doing a search around on the internet, there are two good candidates: Selenium and Cypress, below is a comparison table between them:
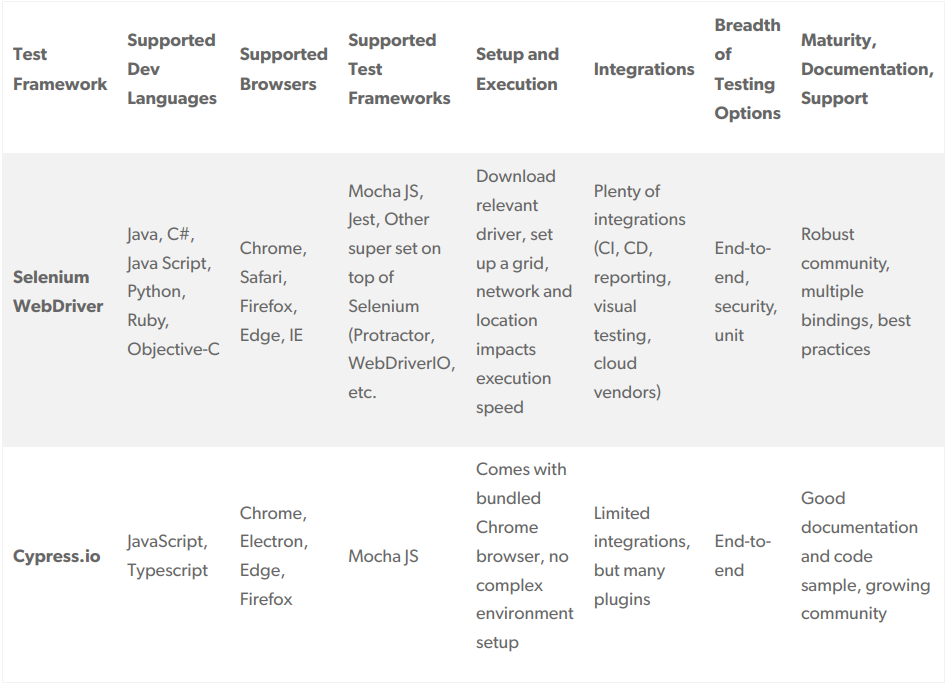
It's easy to figure the Cypress document is more better than Selenium, a clear document helps us approach faster and more accurate so we can learn the best practices from it.
Cypress introduction
- Cypress is an open-source tool which has earned a name for itself in quite a short time for Web integration and End to End UI test automation.
- Cypress is a purely JavaScript-based front end testing tool built for the modern web.Cypress is built on Node.js and comes packed as a npm module.
- Cypress supports Component Testing for the most familiar UI frameworks (React, Vue, Angular, Svelte)
Cypress features
Cypress enables you to write all types of tests:
- End-to-end tests
- Component tests
- Integration tests
- Unit tests
Cypress comes fully baked, batteries included. Here is a list of things it can do that no other testing framework can:
- Time Travel: Cypress takes snapshots as your tests run. Hover over commands in the Command Log to see exactly what happened at each step.
- Debuggability: Stop guessing why your tests are failing. Debug directly from familiar tools like Developer Tools. Our readable errors and stack traces make debugging lightning fast.
- Automatic Waiting: Never add waits or sleeps to your tests. Cypress automatically waits for commands and assertions before moving on. No more async hell.
- Spies, Stubs, and Clocks: Verify and control the behavior of functions, server responses, or timers. The same functionality you love from unit testing is right at your fingertips.
- Network Traffic Control: Easily control, stub, and test edge cases without involving your server. You can stub network traffic however you like.
- Consistent Results: Our architecture doesn’t use Selenium or WebDriver. Say hello to fast, consistent and reliable tests that are flake-free.
- Screenshots and Videos: View screenshots taken automatically on failure, or videos of your entire test suite when run from the CLI. Record to the Dashboard to store them with your test results for zero-configuration debugging.
- Cross browser Testing: Run tests within Firefox and Chrome-family browsers (including Edge and Electron) locally and optimally in a Continuous Integration pipeline.
- Smart Orchestration: Once you're set up to record to the Dashboard, easily parallelize your test suite and rerun failed specs first for tight feedback loops.
- Flake Detection: Discover and diagnose unreliable tests with the Dashboard's Flaky test management.
Cypress architecture
The Node server and browser communication are through the Web Socket, which starts execution after the proxy is created. Cypress sends HTTP requests and responses from the node server to the browser. Cypress works in the network layer; it helps to change the code that might interfere with the automation of the web browser. Cypress interacts with the network layer and captures commands by reading and altering the web traffic.
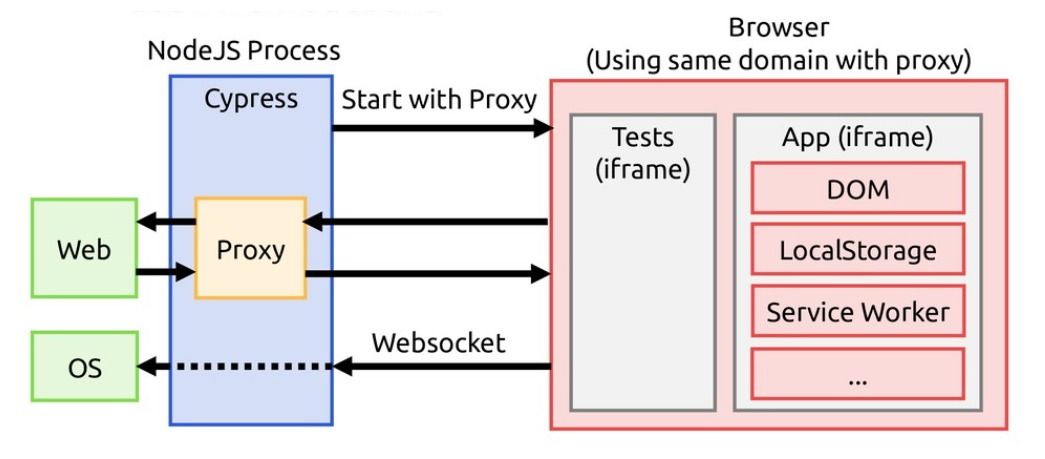
Test spec using Mocha and Chai syntax, it looks like this:
describe('My First Test', () => {
it('clicking "type" navigates to a new url', () => {
cy.visit('https://example.cypress.io')
cy.contains('type').click()
// Should be on a new URL which
// includes '/commands/actions'
cy.url().should('include', '/commands/actions')
})
it('updates the users profile page', function() {
cy.visit('/update_profile')
cy.get('input[name=first_name]').type('Matt')
cy.get('input[name=last_name]').type('Smith')
cy.get('#user-state').select('KY')
cy.get('input[name=address]').type('123 Mission Lane')
cy.get('#phone-number').type('555-123-4567')
cy.get('#zip-code').type('12345')
cy.contains('Save').click()
// we should be redirected to /home and see a “success” message
cy.get('alert-success').should('contain', 'Profile Updated Successfully!')
cy.url().should('include', '/home')
});
})
There are many plugins you can find and apply to your tests available at: https://docs.cypress.io/plugins
Conclusion
Hope you guys have the overview what the Cypress is, I am going to prepare for the Cypress integration with an NextJs application in the next article.
Thanks for reading.
References: