Terraform is a tool that allows users to define infrastruture as code using HCL (HashiCorp Configuration Language). It's a safe and efficient way to build, change, and version infrastructure across multiple cloud providers, such as AWS, Azure, GCP and more.
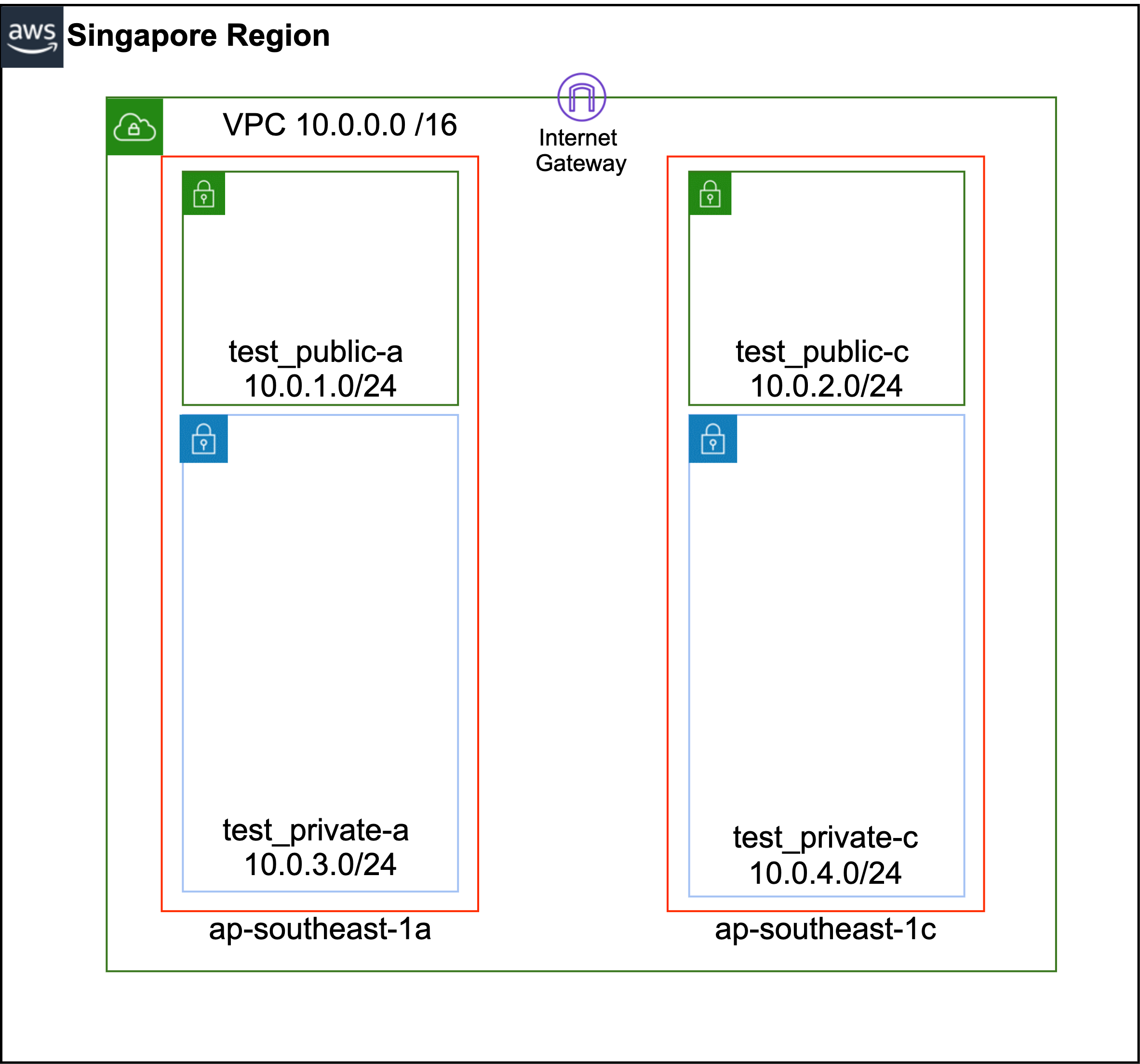
This blog post will guide you through the process of creating a network in the Singapore region using Terraform.

To begin, we need to create two files: variables.tf and vpc.tf.
// variables.tf
variable "az" {
type = list(any)
default = ["ap-southeast-1a", "ap-southeast-1c"]
}
In variables.tf, we'll define a variable called "AZ" for the availability zone and set it to "ap-southeast-1" for the Singapore region.
resource "aws_vpc" "test_vpc" {
cidr_block = "10.0.0.0/16"
enable_dns_hostnames = true
enable_dns_support = true
instance_tenancy = "default"
tags = { Name = "test_vpc" }
}
In vpc.tf, we'll start by defining the VPC CIDR
# subnet public
resource "aws_subnet" "test_public-a" {
vpc_id = aws_vpc.test_vpc.id
cidr_block = "10.0.1.0/24"
availability_zone = var.az[0]
map_public_ip_on_launch = true
tags = {
Name = "test-public-a"
}
}
resource "aws_subnet" "test_public-c" {
vpc_id = aws_vpc.test_vpc.id
cidr_block = "10.0.2.0/24"
availability_zone = var.az[0]
map_public_ip_on_launch = true
tags = {
Name = "test-public-c"
}
}
# subnet private
resource "aws_subnet" "test_private-a" {
vpc_id = aws_vpc.test_vpc.id
cidr_block = "10.0.3.0/24"
availability_zone = var.az[0]
map_public_ip_on_launch = false
tags = {
Name = "test-private-a"
}
}
resource "aws_subnet" "test_private-c" {
vpc_id = aws_vpc.test_vpc.id
cidr_block = "10.0.4.0/24"
availability_zone = var.az[0]
map_public_ip_on_launch = false
tags = {
Name = "test-private-c"
}
}
and create subnets for public and private resources.
Terraform makes it easy to create these subnets with a concise and easy-to-understand configuration.
# igw
resource "aws_internet_gateway" "test_igw" {
vpc_id = aws_vpc.test_vpc.id
tags = {
}
}
We can also configure the Internet gateway
# public routing
resource "aws_route_table" "test_publicRT-a" {
vpc_id = aws_vpc.test_vpc.id
route {
cidr_block = "0.0.0.0/0"
gateway_id = aws_internet_gateway.test_igw.id
}
tags = {
"Name" = "test_publicRT-a"
}
}
resource "aws_route_table" "test_publicRT-c" {
vpc_id = aws_vpc.test_vpc.id
route {
cidr_block = "0.0.0.0/0"
gateway_id = aws_internet_gateway.test_igw.id
}
tags = {
"Name" = "test_publicRT-c"
}
}
# route table association and subnet
resource "aws_route_table_association" "test_routing_public-a" {
subnet_id = aws_subnet.test_public-a.id
route_table_id = aws_route_table.test_publicRT-a.id
}
resource "aws_route_table_association" "test_routing_public-c" {
subnet_id = aws_subnet.test_public-c.id
route_table_id = aws_route_table.test_publicRT-c.id
}
and route table to complete the network setup.
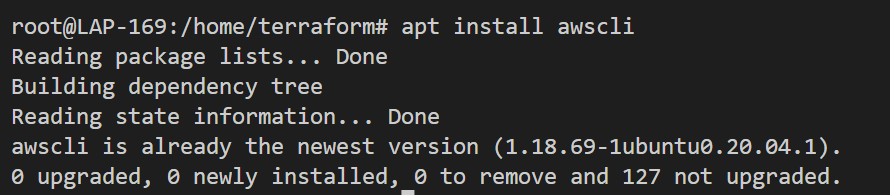
Before applying the Terraform configuration, we need to install the AWS CLI and configure it with our credentials.
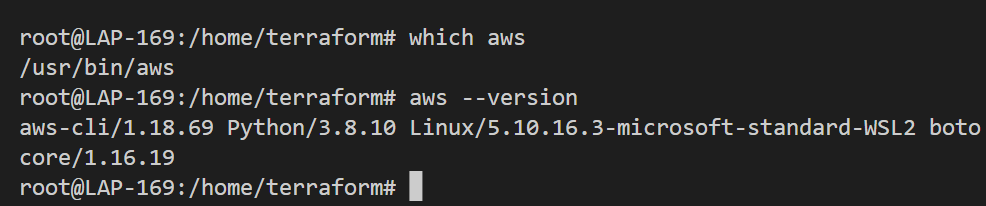
We can confirm this by running the "aws --version" command in our terminal.

To configure our AWS environment, we need to run the "aws configure" command and fill in the access key ID and secret access key for our IAM user, the default region name four our deployment, and the default output format.
$ aws configure
AWS Access Key ID [None] : [ Access Key ID of IAM]
AWS Secret Access Key [None] : [Secret Access Key of IAM]
Default region name [None] : ap-southeast-1 [singapore region]
Default output format [None] :
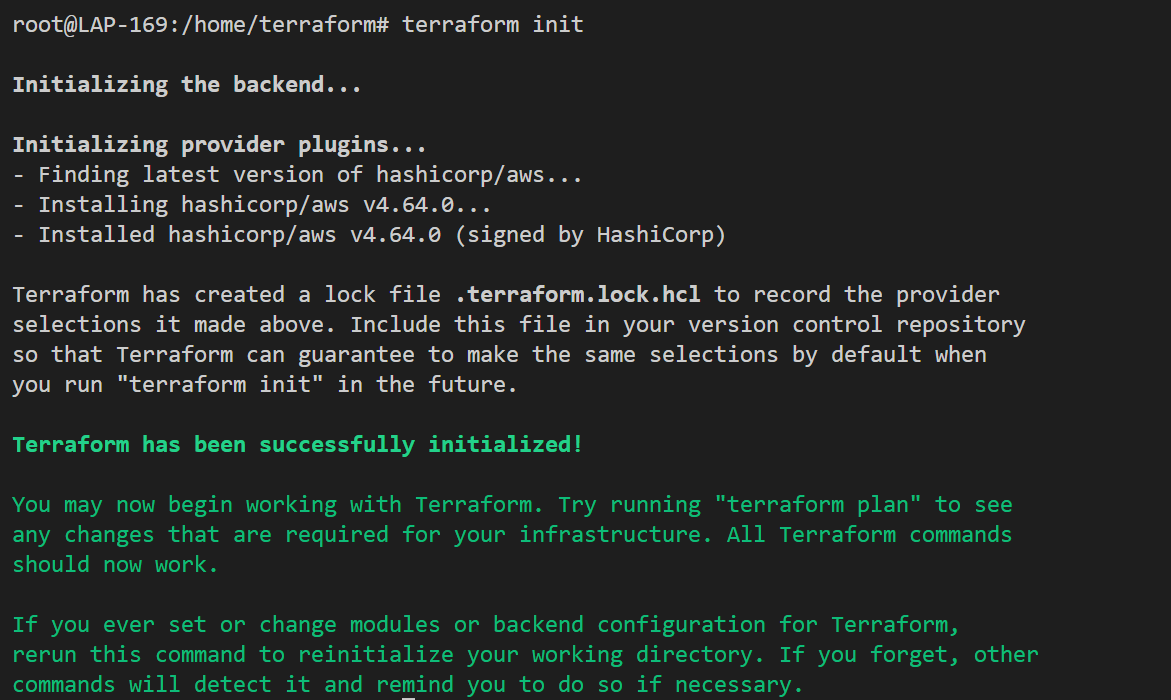
Once everything is set up, we can initialize Terraform with "terraform init" to initialize our Terraform environment
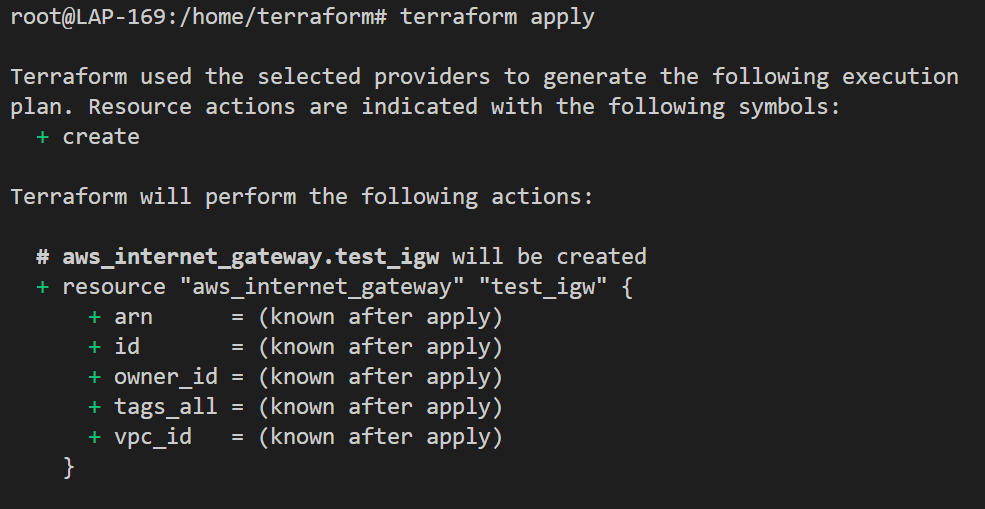

and "terraform apply" to apply our configuration and create our network infrastructure.

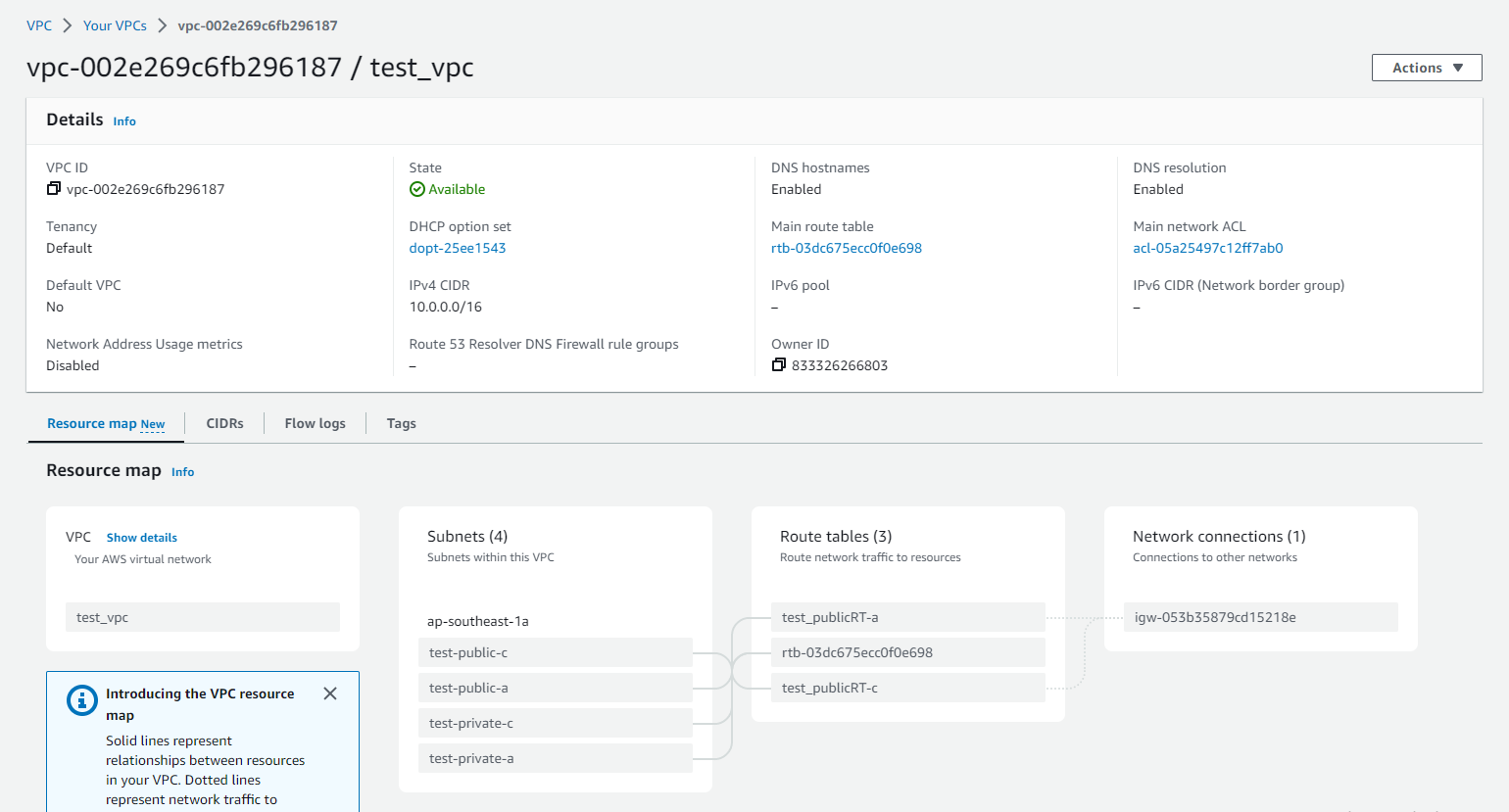
After applying the Terraform configuration, we can verify that our network has been successfully created in the AWS console.


To delete the resources, we can simply run "terraform destroy" to remove all resources created by our Terraform configuration.

And that's it!
Overall, Terraform simplifies infrastructure management by allowing us to easily create and manage resources in the cloud. It's an essential tool for any modern DevOps team.