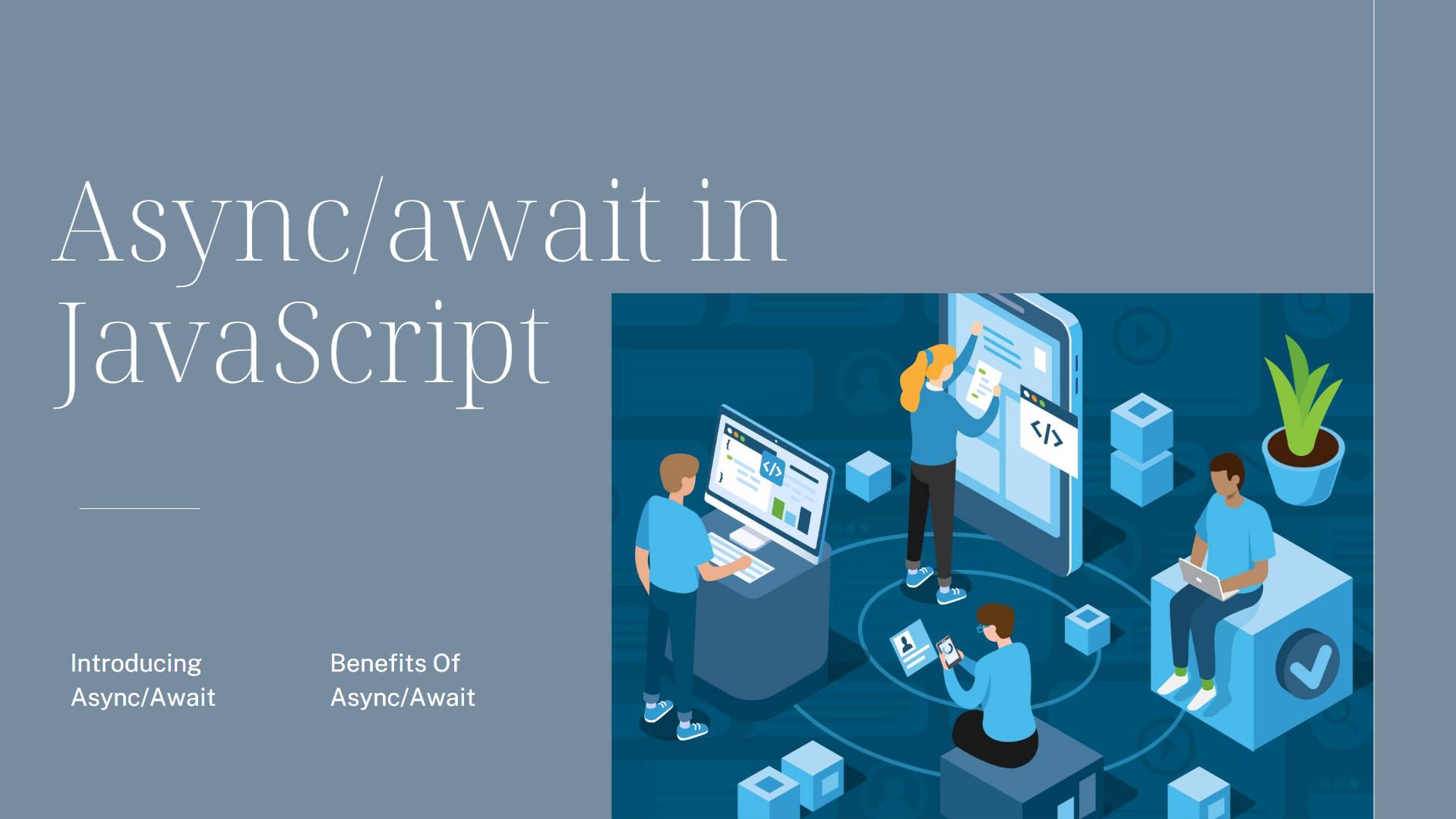
1. Definition
Async/Await is asynchronous code in Javascript, created to simplify the working process.
Async when called to the async function it will handle everything and return the result in its function. Async functions return a Promise, if the function returns an error the promise will be discarded. If the function returns a value the promise will be resolved.
Await is used to wait for a Promise. It can only be used inside an Async block. The Await keyword makes JavaScript wait until the promise returns the result. It should be noted that it only makes the asynchronous function block wait, not the whole program to execute.
2. Syntax
This is the traditional form of callback.
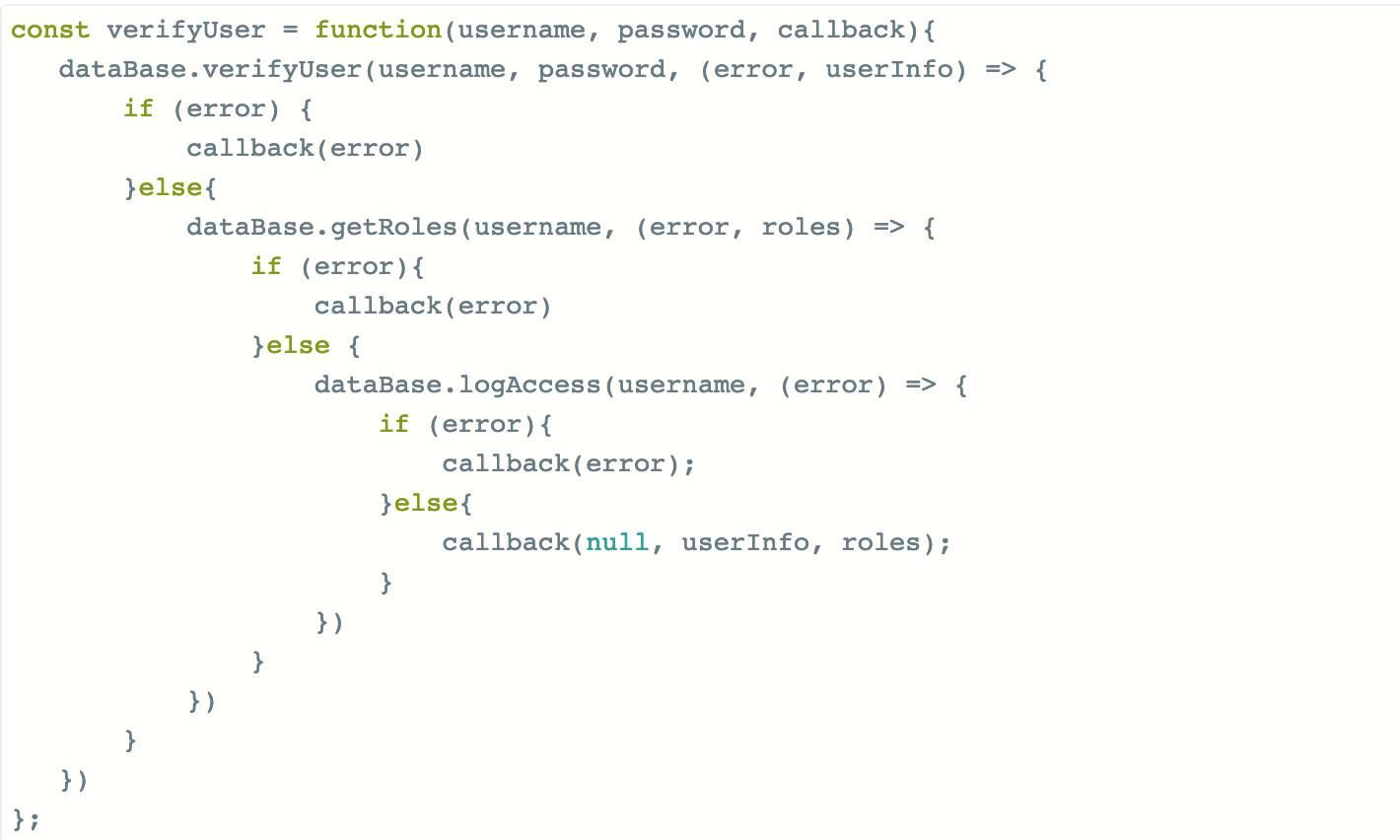
This is Promise

This is Async/Await
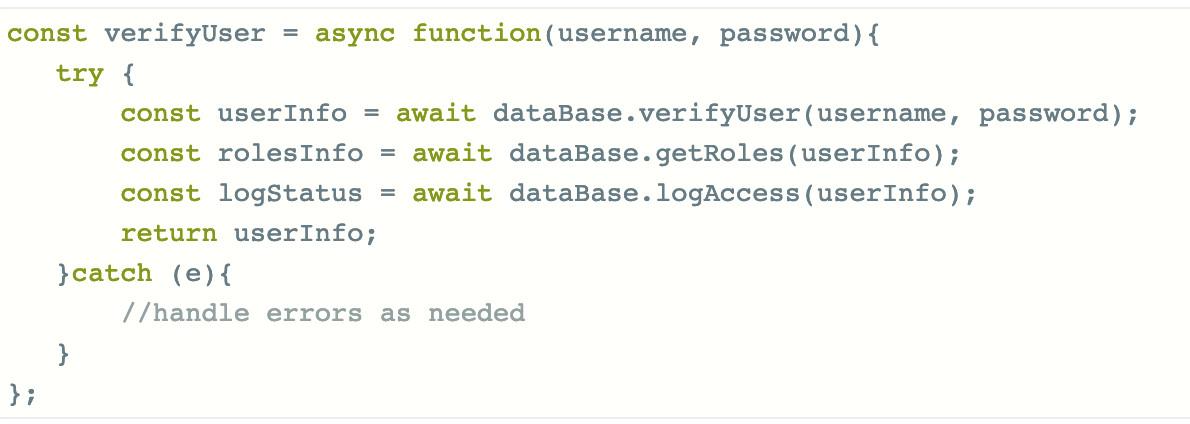
3. Why should we use Async/Await?
The code is easier to read, it's simpler to loop through each element, just await in each loop.
Debugging is easier because each use of await counts as one line of code, so we can set the debugger to debug line by line as usual.
When there is an error, the exception will clearly indicate what line the error is on.
With promises or callbacks, combining if/else or retry with asynchronous code is difficult because we have to write complicated code. With async/await this is much easier.
Below is a basic comparison between Promise and Async/await

4. Summary
Async/Await also has some inadequacies that you need to be aware of when using:
Doesn't work on old browsers. If the project requires to run on old browsers, you will have to use Babel to transpiler code to ES5 to run.
When we await a rejected promise, JavaScript will throw an Exception. Therefore, if you use async await and forget to try catch, when your inconsistent code fails, it can cause your program to stop.
Async and Await must go together! await can only be used in async functions, otherwise you will get a syntax error. Therefore, async/await will spread to all functions in your code.